티스토리 뷰
라우팅 (routing)
📕 주소를 입력해서 들어온 요청을 담당하는 담당자는 함수이고, 요청을 연결하는 작업을 라우팅이라고 한다.
@app.route('/')
def Index():
return 'Index Page'
데코레이터 route
변수 규칙
📕 주소에서 가변적인 부분을 변수라고 한다.
변수를 사용할 땐 <variable_name>처럼 쓰고, 형변환이 필요할 땐 <converter:variable_name> 앞에 자료형을 써준다.
변수를 받아내는 매커니즘이 함수의 매개변수로 전달된다. (변수와 매개변수는 같은 이름이어야 함.)
@app.route('/user/<username>/')
def user_profile(username):
print(username)
return 'User ' + username
@app.route('/post/<int:post_id>/')
def show_post(post_id):
return 'Post ' + post_id
후행 슬래시 (trailing slash)
파일시스템과 비슷하게 / 가 있으면 디렉터리, 없으면 파일을 의미한다.
- URL에 후행 슬래시가 있을 때, 후행 슬래시를 쓰지 않아도 자동으로 붙여서 리다이렉트 한다.
@app.route('/projects/')
def projects():
return 'The project page'
- URL에 후행 슬래시가 없을 때, /about과 /about/은 각각 파일과 디렉터리로 구분하므로 디렉터리로 검색 시 오류가 발생한다.
@app.route('/about')
def about():
return 'The about page'
URL 구축
📕 url_for( )은 url 주소값을 가져오는 함수이다. 매개변수로 함수명을 넣으면 해당 route의 url 주소를 가져온다.
from flask import url_for
@app.route('/')
def Index():
return 'Index Page'
@app.route('/create/')
def create():
return 'Create'
@app.route('/read/<read_id>/')
def read(read_id):
return 'Read '+read_id
with app.test_request_context():
print(url_for('Index')) # /
print(url_for('create')) # /create/
print(url_for('create', next='/')) # /create/?next=%2F
print(url_for('read', read_id=1000)) # /read/1000/
HTTP 메소드
get 방식
📕 데이터가 URL 안에 포함되어있고, URL을 통해 서버를 전송하는 방식 (읽어올 때 씀)
동적 웹서비스에서 특정 페이지를 식별하는 고유한 주소로 사용되는 것이 get 방식 -> 같은 URL를 입력하면 같은 정보를 get할 수 있음
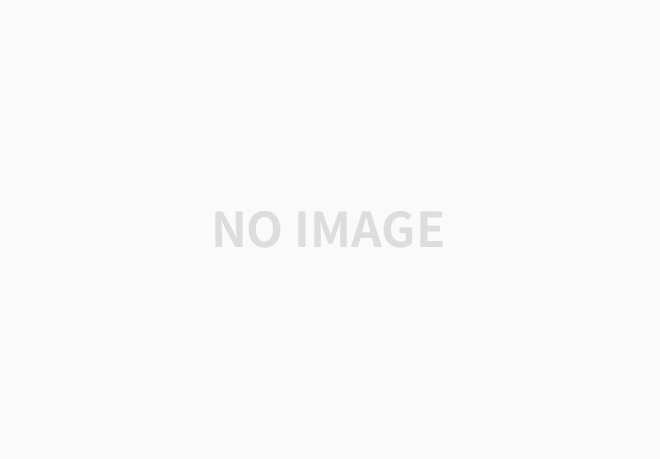
post방식
📕 URL주소에 포함되지 않고 은밀하게 서버로 전송하는 방식 (값을 변경할 때 씀)
변경하려고 하는데 URL에 정보가 있으면 주소로 이동한 순간 정보가 추가되므로 post 방식을 사용
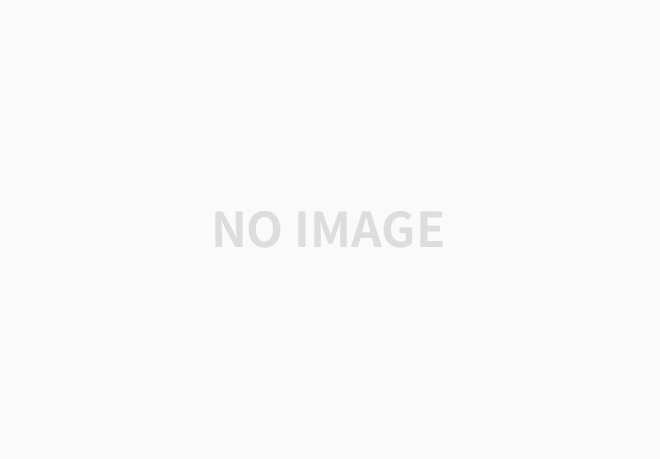
http method
서버로 전송된 데이터가 POST인 경우 route가 허용해줘야 하기 때문에 methods=['GET', 'POST']를 추가한다.
@app.route('/create/', methods=['GET','POST'])
def create():
return f'''
<form action="/create/" method="POST">
<p><input type="text" name="title" placeholder="title"></p>
<p><textarea type="body" name="body" placeholder="body"></textarea></p>
<p><input type="submit" value="create"></p>
</form>
'''
GET과 POST의 출력화면을 다르게 하려면 request를 사용해서 식별해주면 된다.
from flask import request
@app.route('/create/', methods=['GET', 'POST'])
def create():
if request.method == 'POST':
return 'POST'
elif request.method == 'GET':
return 'GET'
참고
https://flask.palletsprojects.com/en/2.1.x/quickstart/#quickstart
'Python > 파이썬 플라스크' 카테고리의 다른 글
[4주차] 로그인/회원가입 처리하기 (0) | 2022.07.18 |
---|---|
[3주차-2] render_template (0) | 2022.07.11 |
[3주차] __init__ / blueprint (0) | 2022.07.11 |
[2주차-2] Python DB API/SQLite3 (0) | 2022.07.08 |
[1주차] 플라스크 개발 환경 설정하기 (0) | 2022.07.03 |